백준 11720 숫자의 합
c++문제풀이
문제
N개의 숫자가 공백 없이 쓰여있다. 이 숫자를 모두 합해서 출력하는 프로그램을 작성하시오.
입력
첫째 줄에 숫자의 개수 N (1 ≤ N ≤ 100)이 주어진다. 둘째 줄에 숫자 N개가 공백없이 주어진다.
출력
입력으로 주어진 숫자 N개의 합을 출력한다.
C++ string
stoi() 함수
접근지정자 활용
ASCII code 활용
- C++ string
#include <iostream>
#include <string>
using namespace std;
int main()
{
int n;
scanf("%d", &n);
string input_str;
getchar(); //while (getchar() != '\n') {} --> clear the buffer
getline(cin, input_str);
string single_str;
int num = 0;
for (int i = 0; i < n; i++)
{
single_str = input_str[i];
num += stoi(single_str);
}
printf("%d", num);
return 0;
}
Defined in header <cstdio>
getchar(): Reads the next character from stdin until EOF
scanf 혹은 cin으로 데이터를 입력받을 때 엔터키 때문에 버퍼에 ‘\n’이 남아있게 된다.
따라서 get char(); 호출하면서 버퍼를 비워준다.
Defined in header <string>
getline(): 공백, 띄어쓰기를 무시하고 ‘\n’까지의 string데이터를 input으로 받는다
stoi(): int stoi( const std::string& str, std::size_t* pos = 0, int base = 10 ) // string to integer from C++11
- 접근지정자 활용
#include <stdio.h>
int main()
{
int n;
scanf("%d", &n);
int value;
int num = 0;
for (int i = 0; i < n; i++)
{
scanf("%1d", &value); //1개씩 입력받음
num += value;
}
printf("%d", num);
return 0;
}
- ASCII code 활용
#include <stdio.h>
int main()
{
int n;
scanf("%d", &n);
int num = 0;
char ch[100];
scanf("%s", ch);
for (int i = 0; i < n; i++)
{
num += ch[i] - '0';
}
printf("%d", num);
return 0;
}
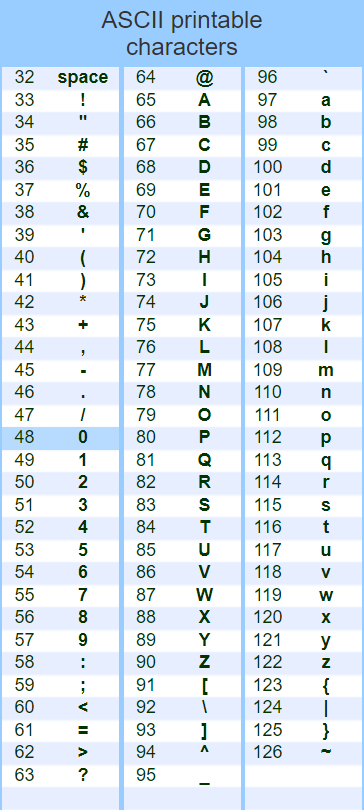
ASCII code 에 대응되는 10진법 값을 활용하여 풀이
‘1’ - ‘0’ = 49 - 48 = 1
‘2’ - ‘0’ = 50 - 48 = 2
‘3’ - ‘0’ = 51 - 48 = 3